import matplotlib.pyplot as plt
import numpy as np
from scipy.integrate import solve_ivp
# constants
= 1.987 # cal⋅/ mol K
RCAL
# data
= 0.02 # cm^2/s
NU_VIS = 0.1 # cm
DP = 10 # cm/s
VEL = 60 # cm^2/g-cat
SP_AREA = 1e-2 # cm^2/s
DE = 10000 # cal/gmol A
DELTA_HR_TR = 25 # cal/gmol K
CPA = 25 # cal/gmol K
CPB = 75 # cal/gmol K -- Solvent is inert
CPS
= 0.01 # cm^3/s g-cat
KA0 = 4000 # cal/mol
EA0 = 300 # K
TR
# Functions
= lambda t: KA0 * np.exp((EA0 / RCAL) * ((1 / TR) - (1 / t)))
k
def reactor(w, y, *args):
# convert dependent variables
= y[0]
x = args
(t0, ca0, fa0, phia, phis, kc)
# isothermal case
= t0
t
# Adiabatic case
= t0 + (x * DELTA_HR_TR / (phia * CPA + phis * CPS))
t
= ca0 * (1-x) * (t0/t)
ca = k(t) * kc * ca / (k(t) + kc)
rate
= rate / fa0
dxdw
= [dxdw]
dydw return dydw
# Problem data
= 1.0e-3 # mol/cm^3
ca0 = 1.0e4 # cm^3/s
v0 = 300
t0 = 1 # 50% A in inlet
phia = 1 # 50% A in inlet
phis
= DP * VEL/ NU_VIS
Re = 100 * Re**0.5
Sh
= Sh * DE/DP # cm/s
kc = kc * SP_AREA # cm^3 /s g-cat
kc
= ca0 * v0
fa0
= (t0, ca0, fa0, phia, phis, kc)
args
= np.array([0])
initial_conditions = 1e6 # 1000 kg
w_final
= solve_ivp(
sol
reactor,0, w_final],
[
initial_conditions,=args,
args="LSODA",
method=True,
dense_output
)
# Extract solution
= np.linspace(0, w_final, 1000)
w
# conversion
= sol.sol(w)[0]
x
# isothermal case
= np.full(len(w), t0)
t
# adiabatic case
= t0 + (x * DELTA_HR_TR / (phia * CPA + phis * CPS)) t
Solutions to workshop 09: External diffusion effects
Lecture notes for chemical reaction engineering
P 14-9
The irreversible gas-phase reaction
\ce{ A ->[ cat ] B}
is carried out adiabatically over a packed bed of solid catalyst particles. The reaction is first order in the concentration of A on the catalyst surface
-r_{As}' = k' C_{As}
The feed consists of 50% (mole) A and 50% inerts, and enters the bed at a temperature of 300 K. The entering volumetric flow rate is 10 dm3/s (i.e., 10,000 cm3/s). The relationship between the Sherwood number and the Reynolds number is
Sh = 100 Re^{1/2}
As a first approximation, one may neglect pressure drop. The entering concentration of A is 1.0 M. Calculate the catalyst weight necessary to achieve 60% conversion of A for
isothermal operation.
adiabatic operation.
Additional information:
- Kinematic viscosity: \mu/\rho = 0.02 cm^2/s
- Particle diameter: d_p = 0.1 cm
- Superficial velocity: U =10 cm/s
- Catalyst surface area/mass of catalyst bed: a= 60 cm^2/g-cat
- Diffusivity of A: D_e = 10^{-2} cm^2/s
- Heat of reaction: \Delta H_{Rx}^{\circ} = 10,000 cal/g mol A
- Heat capacities: C_{pA} = C_{pB} = 25 cal/g mol \cdot K, C_{pS}\, \text{(solvent)} = 75 cal/g mol \cdot K
- k' (300 K) = 0.01 cm^3 /s \cdot g-cat \; \text{with}\; E = 4000 cal/mol
k_c = 4242.641 cm^3/s\ g-cat
C_{A0} = 0.001 mol/cm^3
F_{A0} = 10.000 mol/s
T_0 = 300.000 K
Catalyst weight required for X = 0.6: 538.539 kg
Temperature: 360.08 K
/1000, x, label="conversion")
plt.plot(w0]/1000, w[-1]/1000)
plt.xlim(w[0, 1)
plt.ylim(
plt.grid()
plt.legend()
"weight ($kg$)")
plt.xlabel("Conversion")
plt.ylabel(
plt.show()
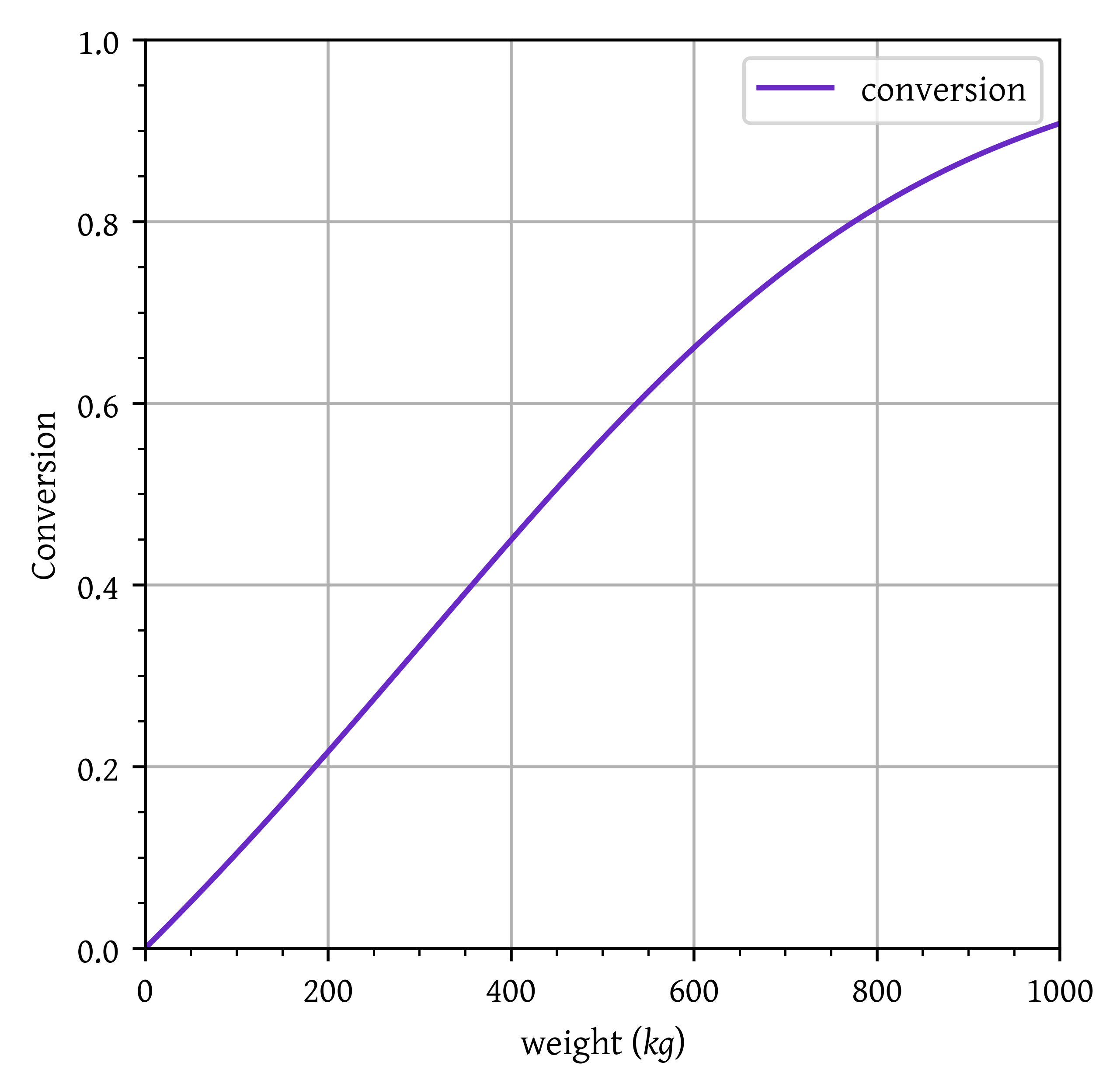
/1000, t, label="temperature")
plt.plot(w0]/1000, w[-1]/1000)
plt.xlim(w[0]-10, t[-1]+10)
plt.ylim(t[
plt.grid()
plt.legend()
"weight ($kg$)")
plt.xlabel("Temperature ($K$)")
plt.ylabel(
plt.show()
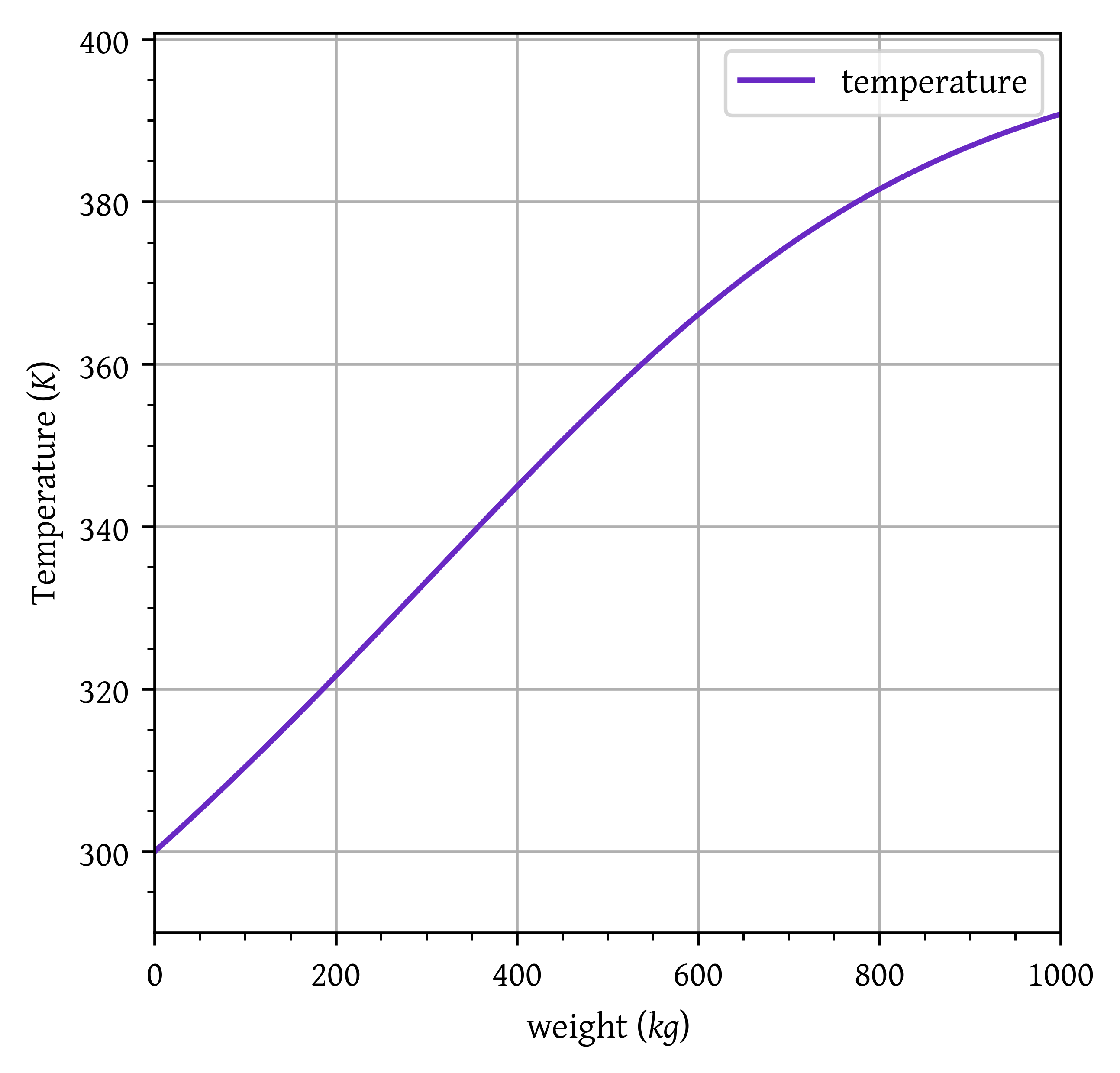
Citation
@online{utikar2024,
author = {Utikar, Ranjeet},
title = {Solutions to Workshop 09: {External} Diffusion Effects},
date = {2024-03-24},
url = {https://cre.smilelab.dev//content/workshops/09-external-and-internal-diffusion-effects/solutions.html},
langid = {en}
}